C.R.U.D
CRUD = Create + Read + Update + Delete
데이터의 생성, 조회, 수정, 삭제
- 컴퓨터 소프트웨어가 가지는 기본적인 데이터 처리 기능인 Create, Read, Update, Delete를 묶어서 말하는 것이다.
- DB SQL문과 대응된다. Create-Insert / Read-Select / Update-Update / Delete-Delete
Prisma Client
prisma로 연결되어 있는 db의 table들을 쉽게 접근할 수 있게 해주는 client역할.
prisma.schema 파일에서 정의한 table,col 이름을 직접 사용할 수 있게 함.
설치
npm install @prisma/client --save
prisma generate
: prisma.schema파일을 읽어서 이 파일에 있는 모델 형태에 맞는 client생성
: schema변경할 때 마다 client API 변경사항 수용위해 prisma generate로 수동호출
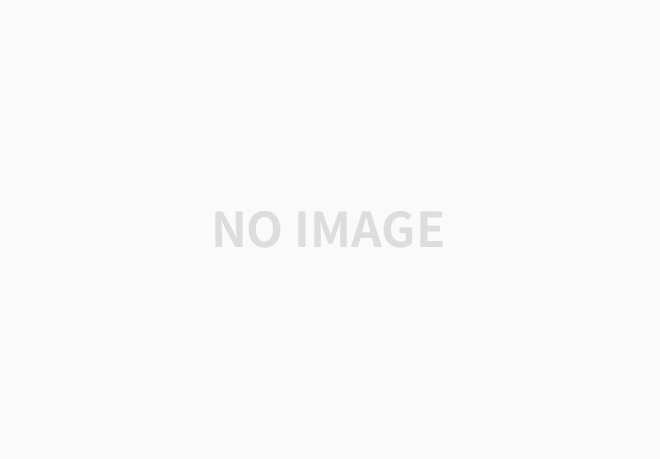
ORM
: object-relational mapping (db에 있는 데이터를 하나의 객체에 매핑)
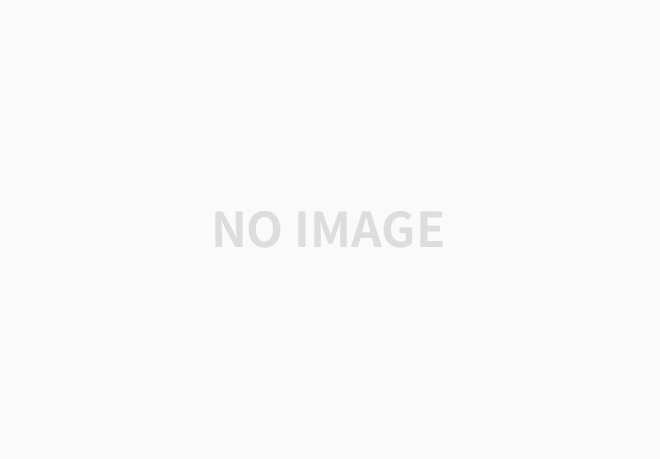
Create
Create a single record
( ex 회원가입, 좋아요 기능)
#create a single user with two fields
const user = await prisma.user.create({
data: {
email: 'elsa@prisma.io',
name: 'Elsa Prisma',
},
})
#The user's id is auto-generated, and your schema determines which fields are mandatory
Create multiple records
const createMany = await prisma.user.createMany({
data: [
{ name: 'Bob', email: 'bob@prisma.io' },
{ name: 'Bobo', email: 'bob@prisma.io' }, // Duplicate unique key!
{ name: 'Yewande', email: 'yewande@prisma.io' },
{ name: 'Angelique', email: 'angelique@prisma.io' },
],
skipDuplicates: true, // Skip 'Bobo'
})
Raw Query
app.post('/categories', async (req, res) => {
const category = await prisma.$queryRaw`
INSERT INTO categories (name) #table이름(매핑 후)
VALUES ('콜드 브루'); #sql문이니까 세미콜론
`;
res.json(category); // []
});
app.post('/categories', async (req, res) => {
await prisma.$queryRaw`
INSERT INTO categories (name)
VALUES ('콜드 브루');
`;
const [category] = await prisma.$queryRaw` #ORM과 같이 해주기 위해서 추가
#SELECT * #최근 추가한 데이터를 반환
SELECT c.id, c.name #category를 줄여서
FROM categories c
ORDER BY id DESC #아이디 뒤집어서첫번째꺼 (추가된거)
LIMIT 1
`;
res.json(category);
});
> queryRaw 매서드는 기본적으로 배열로반환
> const category를 [category]를 객체로 반환(하나일떄) - 배열비구조화
> select * 전부다 불러옴
Read
Get record by ID or unique identifier
( ex 상품리스트 정보, 상품 디테일 정보)
Return a single record (findUnique ) by unique identifier or ID:
// By unique identifier
const user = await prisma.user.findUnique({
where: {
email: 'elsa@prisma.io',
},
})
// By ID
const user = await prisma.user.findUnique({
where: {
id: 99,
},
})
Get all records
const users = await prisma.user.findMany()
ex)
// id가 1인 음료 불러오기
app.get('/drinks/:id', async (req, res) => {
const { id } = req.params;
const drink = await prisma.drink.findUnique({
where: {
id: +id,
},
});
res.json(drink);
});
//where에 id는 number type들어가야하는데 params에서 가져온 id는 string이라 타입변환
전부
//전부
app.get('/drinks', async (req, res) => {
const allDrinks = await prisma.$queryRaw`
SELECT d.id, d.name, d.category_id, d.korean_name, d.english_name, d.description, d.is_new
FROM drinks d
`;
res.json(allDrinks);
});
Update
ex) 회원 정보 수정
app.put('/users', async (req, res) => {
const { id } = req.query;
const { email, password } = req.body;
const updateUser = await prisma.user.update({
where: { id}, //조건 (여기서는 req보내주는 id랑 동일했을 때)
data: { email, password,} //새로 업데이트 할 column 명을 객체형태로 넣고 새로운 값 수정
});
res.json(updateUser);
});
// raw query
app.put('/users', async (req, res) => {
const { id } = req.query;
const { email, password } = req.body;
const updateUser = await prisma.$queryRaw`
UPDATE users //업데이트 할 테이블 이름
SET email=${email}, password=${password} //업데이트 할 컬럼과 값
WHERE id=${id}; //입력안하면 모든 데이터 업데이트
`;
res.json(updateUser);
});
Delete
ex) 좋아요 삭제, 유저 정보 삭제
app.delete('/users', async (req, res) => {
const { id } = req.query;
const deletedUserCount = await prisma.user.deleteMany({
where: { id }
});
res.json(deletedUserCount);
});
app.delete('/users', async (req, res) => {
const { id } = req.query;
const deletedUserCount = await prisma.$queryRaw`
DELETE FROM users
WHERE id=${id}
`
res.json(deletedUserCount);
});
'Archive' 카테고리의 다른 글
[JS] 삼항연산 / spread / Optional Chaining / Destructuring (0) | 2021.09.22 |
---|---|
[JS] querySelector (0) | 2021.09.18 |
[DB] Database 기초 (0) | 2021.09.14 |
[Node.js] express (0) | 2021.09.13 |
[Node.js] Protocol / Port / URL (0) | 2021.09.13 |